从 flutter_driver 迁移
本页面介绍如何迁移使用 flutter_driver
的现有项目到 integration_test
包,以便运行集成测试。
使用 integration_test
的测试使用与Widget 测试中相同的方法。
有关 integration_test
包的介绍,请查看集成测试指南。
入门示例项目
#本指南中的项目是一个具有以下功能的小型桌面应用程序示例
- 左侧是用户可以滚动、点击和选择植物的列表。
- 右侧是显示植物名称和种类的详细信息屏幕。
- 应用程序启动时,如果未选择任何植物,则会显示一条文本,提示用户选择植物。
- 植物列表从位于
/assets
文件夹中的本地 JSON 文件加载。
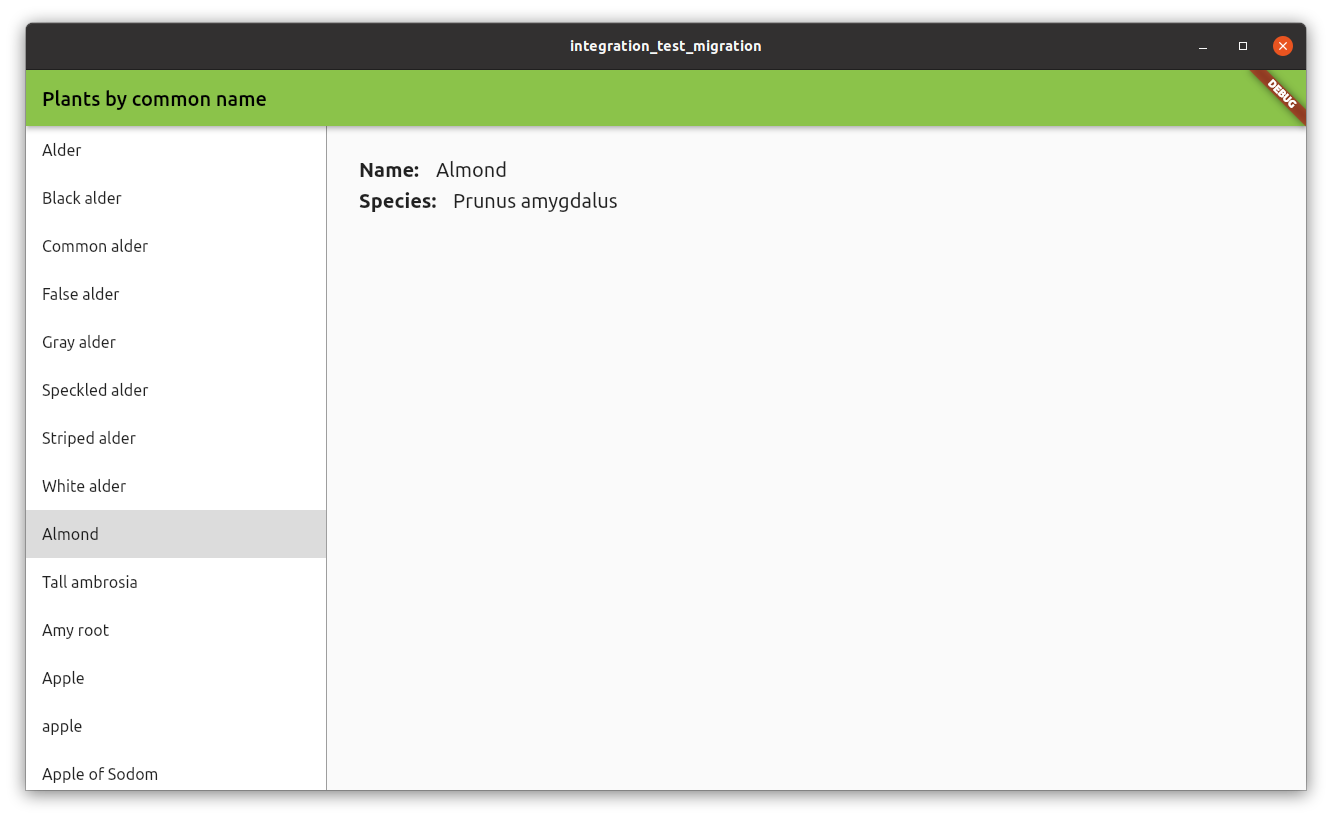
您可以在示例项目文件夹中找到完整的代码示例。
现有测试
#该项目包含三个执行以下检查的 flutter_driver
测试
- 验证应用程序的初始状态。
- 选择植物列表中的第一个项目。
- 滚动并选择植物列表中的最后一个项目。
这些测试包含在 test_driver
文件夹内的 main_test.dart
文件中。
在此文件夹中还有一个名为 main.dart
的文件,其中包含对 enableFlutterDriverExtension()
方法的调用。使用 integration_test
后,此文件将不再需要。
设置
#要开始使用 integration_test
包,请将 integration_test
添加到您的 pubspec.yaml
文件中(如果您尚未添加)。
dev_dependencies:
integration_test:
sdk: flutter
接下来,在您的项目中,创建一个名为 integration_test/
的新目录,并在其中创建您的测试文件,格式为:<name>_test.dart
。
测试迁移
#本节包含有关如何将现有的 flutter_driver
测试迁移到 integration_test
测试的不同示例。
示例:验证 Widget 是否显示
#应用程序启动时,右侧屏幕会显示一条文本,提示用户从列表中选择一种植物。
此测试验证该文本是否显示。
flutter_driver
在 flutter_driver
中,测试使用 waitFor
,它等待直到 finder
可以定位 Widget。如果找不到 Widget,则测试将失败。
test('do not select any item, verify please select text is displayed',
() async {
// Wait for 'please select' text is displayed
await driver.waitFor(find.text('Please select a plant from the list.'));
});
integration_test
在 integration_test
中,您需要执行两个步骤
首先使用
tester.pumpWidget
方法加载主应用程序 Widget。然后,使用
expect
和匹配器findsOneWidget
验证 Widget 是否显示。
testWidgets('do not select any item, verify please select text is displayed',
(tester) async {
// load the PlantsApp widget
await tester.pumpWidget(const PlantsApp());
// wait for data to load
await tester.pumpAndSettle();
// Find widget with 'please select'
final finder = find.text('Please select a plant from the list.');
// Check if widget is displayed
expect(finder, findsOneWidget);
});
示例:点击操作
#此测试对列表中的第一个项目执行点击操作,该项目是文本为“Alder”的 ListTile
。
点击后,测试将等待详细信息出现。在这种情况下,它将等待文本为“Alnus”的 Widget 显示。
此外,测试还验证文本“Please select a plant from the list.” 是否不再显示。
flutter_driver
在 flutter_driver
中,使用 driver.tap
方法使用 finder 对 Widget 执行点击操作。
要验证 Widget 是否未显示,请使用 waitForAbsent
方法。
test('tap on the first item (Alder), verify selected', () async {
// find the item by text
final item = find.text('Alder');
// Wait for the list item to appear.
await driver.waitFor(item);
// Emulate a tap on the tile item.
await driver.tap(item);
// Wait for species name to be displayed
await driver.waitFor(find.text('Alnus'));
// 'please select' text should not be displayed
await driver
.waitForAbsent(find.text('Please select a plant from the list.'));
});
integration_test
在 integration_test
中,使用 tester.tap
执行点击操作。
在点击操作后,必须调用 tester.pumpAndSettle
以等待操作完成,以及所有 UI 更改都发生。
要验证 Widget 是否未显示,请使用相同的 expect
函数和 findsNothing
匹配器。
testWidgets('tap on the first item (Alder), verify selected', (tester) async {
await tester.pumpWidget(const PlantsApp());
// wait for data to load
await tester.pumpAndSettle();
// find the item by text
final item = find.text('Alder');
// assert item is found
expect(item, findsOneWidget);
// Emulate a tap on the tile item.
await tester.tap(item);
await tester.pumpAndSettle();
// Species name should be displayed
expect(find.text('Alnus'), findsOneWidget);
// 'please select' text should not be displayed
expect(find.text('Please select a plant from the list.'), findsNothing);
});
示例:滚动
#此测试类似于之前的测试,但它向下滚动并点击最后一个项目。
flutter_driver
要使用 flutter_driver
向下滚动,请使用 driver.scroll
方法。
您必须提供要执行滚动操作的 Widget,以及滚动的持续时间。
您还必须提供滚动操作的总偏移量。
test('scroll, tap on the last item (Zedoary), verify selected', () async {
// find the list of plants, by Key
final listFinder = find.byValueKey('listOfPlants');
// Scroll to the last position of the list
// a -100,000 pixels is enough to reach the bottom of the list
await driver.scroll(
listFinder,
0,
-100000,
const Duration(milliseconds: 500),
);
// find the item by text
final item = find.text('Zedoary');
// Wait for the list item to appear.
await driver.waitFor(item);
// Emulate a tap on the tile item.
await driver.tap(item);
// Wait for species name to be displayed
await driver.waitFor(find.text('Curcuma zedoaria'));
// 'please select' text should not be displayed
await driver
.waitForAbsent(find.text('Please select a plant from the list.'));
});
integration_test
使用 integration_test
,可以使用 tester.scrollUntilVisible
方法。
无需提供要滚动的 Widget,只需提供要搜索的项目即可。在这种情况下,您正在搜索文本为“Zedoary”的项目,它是列表中的最后一个项目。
该方法搜索任何 Scrollable
Widget 并使用给定的偏移量执行滚动操作。该操作会重复执行,直到项目可见。
testWidgets('scroll, tap on the last item (Zedoary), verify selected',
(tester) async {
await tester.pumpWidget(const PlantsApp());
// wait for data to load
await tester.pumpAndSettle();
// find the item by text
final item = find.text('Zedoary');
// finds Scrollable widget and scrolls until item is visible
// a 100,000 pixels is enough to reach the bottom of the list
await tester.scrollUntilVisible(
item,
100000,
);
// assert item is found
expect(item, findsOneWidget);
// Emulate a tap on the tile item.
await tester.tap(item);
await tester.pumpAndSettle();
// Wait for species name to be displayed
expect(find.text('Curcuma zedoaria'), findsOneWidget);
// 'please select' text should not be displayed
expect(find.text('Please select a plant from the list.'), findsNothing);
});
除非另有说明,否则本网站上的文档反映了 Flutter 的最新稳定版本。页面上次更新于 2024-05-03。 查看源代码 或 报告问题.